Merry Christmas !
Drawing a Christmas tree in C can be achieved by printing a character pattern to the console. Below is a simple C program example that uses * and space characters to print a Christmas tree
#include <stdio.h>
int main() {
int height = 10; // Set the height of the tree
for (int i = 1; i <= height; i++) {
// Print spaces to center the tree
for (int j = i; j < height; j++) {
printf(" ");
}
// Print stars to form the tree
for (int k = 0; k < (2 * i - 1); k++) {
printf("*");
}
// Move to the next line after each row
printf("\n");
}
// Print the tree trunk
for (int i = 0; i < 2; i++) { // Tree trunk height
for (int j = 0; j < height - 1; j++) {
printf(" "); // Align the trunk with the tree
}
printf("|\n"); // Print the trunk
}
return 0;
}
CExplanation:
- Tree Structure:
- The outer loop (
for (int i = 1; i <= height; i++)
) controls the rows of the tree. - Spaces are printed first (
for (int j = i; j < height; j++)
) to center-align the tree. - Stars are printed (
for (int k = 0; k < (2 * i - 1); k++)
) to form the triangular shape of the tree.
- The outer loop (
- Tree Trunk:
- The trunk is created using a fixed number of rows (
for (int i = 0; i < 2; i++)
). - Spaces are printed before the trunk to align it with the tree.
- The trunk is represented by a vertical bar (
|
).
- The trunk is created using a fixed number of rows (
- Customization:
- You can change the
height
variable to adjust the size of the tree. - Modify the trunk’s height or width by adjusting the corresponding loops.
- You can change the
Colorful Christmas Tree in C with Random Ornaments
#include <stdio.h>
#include <stdlib.h> // For rand(), srand()
#include <time.h> // For time()
// ANSI color codes
#define RESET "\033[0m"
#define GREEN "\033[32m"
#define RED "\033[31m"
#define YELLOW "\033[33m"
#define CYAN "\033[36m"
// Function to draw a colorful Christmas tree
void draw_colored_tree(int height) {
for (int i = 1; i <= height; i++) {
// Print spaces for alignment to center the tree
for (int j = i; j < height; j++) {
printf(" ");
}
// Print stars with random colors for ornaments
for (int k = 0; k < (2 * i - 1); k++) {
if (rand() % 3 == 0) {
printf(RED "*"); // Random red ornaments
} else if (rand() % 3 == 1) {
printf(YELLOW "*"); // Random yellow ornaments
} else {
printf(GREEN "*"); // Default green tree
}
}
// Move to the next line
printf(RESET "\n");
}
// Draw the trunk with cyan color
for (int i = 0; i < 2; i++) { // Trunk height (fixed at 2)
for (int j = 0; j < height - 1; j++) {
printf(" ");
}
printf(CYAN "|\n" RESET); // Cyan-colored trunk
}
}
int main() {
int height = 25; // Height of the tree
// Seed random number generator
srand(time(NULL));
// Draw the colorful Christmas tree
draw_colored_tree(height);
return 0;
}
CExplanation:
- Tree Height:
- The tree will be 25 rows tall, as specified by the
height = 25;
in themain
function.
- The tree will be 25 rows tall, as specified by the
- Random Colored Ornaments:
- Each
*
(star) in the tree is colored randomly usingrand() % 3
:- Red (
\033[31m
), Yellow (\033[33m
), or Green (\033[32m
).
- Red (
- Each
- Tree Trunk:
- The trunk is printed at the bottom of the tree with a height of 2 lines, using the cyan color (
\033[36m
).
- The trunk is printed at the bottom of the tree with a height of 2 lines, using the cyan color (
- Color Reset:
- After each line of stars and after the trunk, the
RESET
code (\033[0m
) is used to restore the default console color.
- After each line of stars and after the trunk, the
Example Output:
The tree will appear with stars in random colors and a cyan trunk, like this (though your output will have different star colors every time due to randomness):
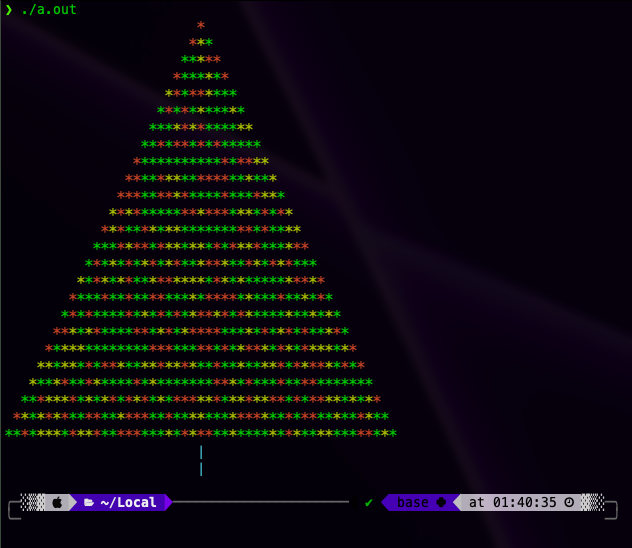
Leave a Reply